
| 
How to call an Integra profile after submitting a document from the Web browser
Let us take the following scenario. A user is creating a Notes document and after filling in the required information, needs to generate a report using Integra for Notes. It is possible to trigger an Integra profile from the onUnload or WebQuerySave event of the document.
Below are three examples of how this can be implemented:
- Calling Integra from the onUnload event of the Notes document
- Calling an agent from the WebQuerySave event of the Notes document
- Triggering the agent which processes scheduled profiles in the Integra database
In the first two examples, when the Integra profile is triggered, the user will be presented with the Processing dialog until the Integra profile execution is completed. In the third example, the Integra profile is executed on the server, therefore without any user interface.
The following needs to be done for both examples:
- Create a field "IsSubmitted" on the form having a default value of ""
- Create a button "Submit" on the form and place the following code in the onClick event:
var frm = window.document.forms[0];
//Set the field IsSubmitted to 1 to indicate that the form has
//been submitted from the button and therefore
//has not been submitted from another process, such as a field refresh
frm.IsSubmitted.value = "1";
frm.submit();
- Refer to example A or B below according to the scenario you would like to implement.
A. Calling Integra from the onUnload event of the Notes document
Place the following code in the onUnload event of the form and amend as per the comments:
var frm = window.document.forms[0];
//Do not trigger the Integra profile if the form has not
//been submitted from the Submit button
if (frm.IsSubmitted.value != "1")
return;
// Enter name of the Integra profile to be executed
var ProfileName = "enter the profile name here";
//The profile will be executed on the current document
var UseCurrentDoc = 1;
//Error message displayed when cookies are disabled
//You can modify this to display different messages depending on the language
//selected by the user
var errmsg = "Integra for Notes cannot run because cookies are disabled!";
//=============================================
// Do not change the code below !!!!!!
if (window.navigator.cookieEnabled == false)
{
alert(errmsg);
}
else
{
var url = window.location;
var path = url.pathname.toLowerCase();
var dbpos = path.indexOf(".nsf");
var dtExpires = new Date((new Date()).getTime() + 1000 * 60);
var cCooking;
cCooking = "IntegraProfileName=" + escape(ProfileName); // The name=value
cCooking += "; expires=" + dtExpires.toGMTString();
window.document.cookie = cCooking;
cCooking = "IntegraCurrentDoc=" + UseCurrentDoc; // The name=value
cCooking += "; expires=" + dtExpires.toGMTString();
window.document.cookie = cCooking;
path = path.substring(0,dbpos+5)+"Integra4WebSelectedDocuments?Openform";
var wwidth = 550;
var wheight = 325;
var scr = window.screen;
var top = (scr.width-wwidth)/2;
var left = (scr.height-wheight)/2;
window.open(path, "", "width=" + wwidth + ",height=" + wheight +
",left=" + left + ",top=" + top +
",toolbar=0,location=0,status=0,scrollbars=0,
resizable=0,menubar=0,directories=0");
}
B. Calling an agent from the WebQuerySave event of the Notes document
- Create an agent which has settings enclosed in the red box in the screenshot below:
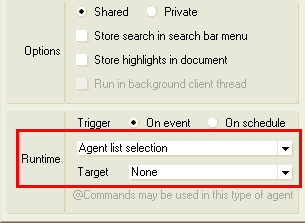
- Call this agent from the WebQuerySave event of the Notes document.
- Place the following code in the agent's Initialize event:
Sub Initialize
On Error Goto errhandle
Dim session As New NotesSession
Dim doc As NotesDocument
Set doc = session.DocumentContext
'Do not trigger the Integra profile if the form has
'not been submitted from the Submit button
If Not doc.IsSubmitted(0) = "1" Then
Exit Sub
End If
Print | <SCRIPT LANGUAGE='JavaScript'>
try{
// Enter name of the Integra profile to be executed
var ProfileName = "enter the profile name here";
//The profile will be executed on the current document
var UseCurrentDoc = 1;
//Error message displayed when cookies are disabled
//You can modify this to display different messages depending on the language
//selected by the user
var errmsg = "Integra for Notes cannot run because cookies are disabled!";
//=============================================
// Do not change the code below !!!!!!
if (window.navigator.cookieEnabled == false)
{
alert(errmsg);
}
else
{
var url = window.location;
var path = url.pathname.toLowerCase();
var dbpos = path.indexOf(".nsf");
var dtExpires = new Date((new Date()).getTime() + 1000 * 60);
var cCooking;
cCooking = "IntegraProfileName=" + escape(ProfileName); // The name=value
cCooking += "; expires=" + dtExpires.toGMTString();
window.document.cookie = cCooking;
cCooking = "IntegraCurrentDoc=" + UseCurrentDoc; // The name=value
cCooking += "; expires=" + dtExpires.toGMTString();
window.document.cookie = cCooking;
path = path.substring(0,dbpos+5)+"Integra4WebSelectedDocuments?Openform";
var wwidth = 550;
var wheight = 325;
var scr = window.screen;
var top = (scr.width-wwidth)/2;
var left = (scr.height-wheight)/2;
window.open(path, "", "width=" + wwidth + ",height=" + wheight +
",left=" + left + ",top=" + top +
",toolbar=0,location=0,status=0,scrollbars=0,
resizable=0,menubar=0,directories=0");
}
} catch(e) {
alert(e.message);
}
</SCRIPT> |
NormalExit:
Exit Sub
Errhandle:
Print Erl() & ": " & Err() & ", " & Error()
Resume NormalExit
End Sub
C. Triggering the agent which processes scheduled profiles in the Integra database
- Create an agent which has settings enclosed in the red box in the screenshot below:
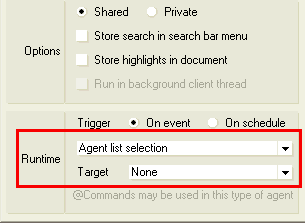
- Call this agent from the WebQuerySave event of the Notes document.
- Place the following code in the agent's Initialize event:
Sub Initialize
On Error Goto Errhandle
Dim session As New NotesSession
Dim db As New NotesDatabase("","")
Dim vw As NotesView
Dim agent As NotesAgent
Dim doc As NotesDocument
Dim srv As String
Dim repid As String
srv = session.Currentdatabase.Server
repid = session.GetEnvironmentString("IntegraReplicaID")
repid = Left(repid,8) & Right(repid,8)
'Open the Integra database
If Not db.OpenByReplicaID(srv,repid) Then
Print "Unable to open the Integra database"
End If
'Get a handle to the Integra profile to be processed
Set vw = db.GetView("Import/Export")
'Enter name of the Integra profile to be executed
Set doc = vw.GetDocumentByKey("enter the profile name here")
'Flag the Integra profile to be processed immediately
doc.ProcessingStatus = "Run Now (one time only)"
Call doc.save(True,False,False)
'Trigger the agent which processed scheduled Integra profiles
Set agent = db.GetAgent("Import/Export For Scheduled Profile")
Call agent.RunOnServer()
NormalExit:
Exit Sub
Errhandle:
Print Erl() & ": " & Err() & ", " & Error()
Resume NormalExit
End Sub
| 
. |